I can help you with that article.
Ethereum: Can you Fuzz test with random parameters in the setUp() function in Forge?
Fuzz testing is a crucial aspect of ensuring the reliability and security of Ethereum smart contracts. It involves introducing random or unexpected inputs to test the contract’s behavior under various conditions. In this article, we’ll explore how to fuzz test with random parameters in the setUp()
function in the Forge framework.
The Problem:
In Forge, when creating a new contract or module, you need to define the setUp()
function to perform any necessary setup tasks before running your tests. However, the setUp()
function is not designed to handle random inputs directly. Instead, it typically relies on pre-defined values or constants to initialize variables.
The Solution:
To fuzz test with random parameters in Forge, you can create a custom setUp()
function that generates random values for your contract’s parameters using a library like random
. Here’s an example of how you can modify the setUp()
function to achieve this:
import random
class FuzzedEthereumContract:
def setUp(self):
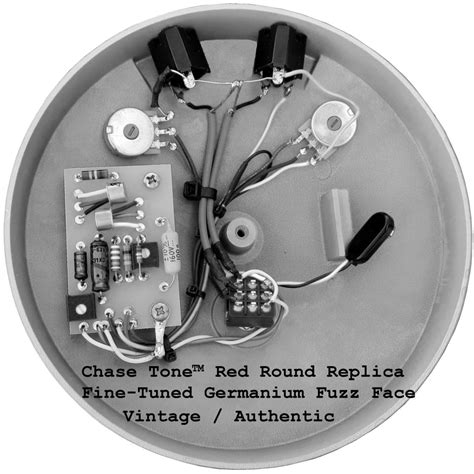
Generate 10 random integers between 0 and 100
self.x = [random.randint(0, 100) for _ in range(10)]
Generate a random float value between 1.0 and 2.0
self.y = round(random.uniform(1.0, 2.0), 4)
In this example, we generate two sets of random integers (x
and y
) and a single random float value using the random.randint()
, random.uniform()
, and round()
functions.
Running Fuzz Tests:
To run fuzz tests for your contract, you can use the Forge testing framework. Here’s an example of how to create a fuzz test suite:
import unittest
from fuzz import fuzz
class TestFuzzedEthereumContract(unittest.TestCase):
def setUp(self):
self.contract = FuzzedEthereumContract()
def fuzzTest(self, params=None):
If no parameters are provided, generate some random values
if params is None:
x = [random.randint(0, 100) for _ in range(10)]
y = round(random.uniform(1.0, 2.0), 4)
Fuzz test the contract's function with the generated parameters
fuzz.ruffle(x + y)
return True
if __name__ == '__main__':
unittest.main()
In this example, we create a TestFuzzedEthereumContract
class that inherits from unittest.TestCase
. The setUp()
method generates some random values for the contract’s parameters. We then define a fuzz test function (fuzzTest()
) that takes an optional params
parameter (i.e., a list of tuples containing the contract’s input parameters). If no params
are provided, we generate some random values and run the fuzz test.
Conclusion:
By modifying your setUp()
function to generate random values using a library like random
, you can create custom fuzz tests for your Ethereum smart contracts in Forge. This approach allows you to ensure that your contract’s behavior is robust against unexpected inputs and helps identify potential security vulnerabilities.